Get Started with MicroPython and the Raspberry Pi Pico
A step-by-step guide for programming your Raspberry Pi Pico using MicroPython, from setting up the environment to writing your first program. …
Updated September 3, 2023
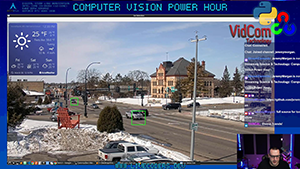
Need help with your Raspberry Pi?
Contact Me!
Do you love silly Raspberry Pi Projects?
Check out my this YouTube Channel!
A step-by-step guide for programming your Raspberry Pi Pico using MicroPython, from setting up the environment to writing your first program.
Are you looking to learn how to program a Raspberry Pi Pico? This tutorial will provide you with a step-by-step guide on getting started with MicroPython and programming your Raspberry Pi Pico. MicroPython is a Python-based programming language that allows for easy development and prototyping of embedded systems, such as the Raspberry Pi Pico.
Setting up the Environment
Download the MicroPython UF2 file from https://www.raspberrypi.com/software/
Connect your Raspberry Pi Pico to your computer via a USB cable. The green LED should light up when it’s connected.
Double-click on the downloaded UF2 file to flash MicroPython onto your Raspberry Pi Pico. This process may take a few seconds and will automatically mount the device as a drive named ‘RPI-RP2’.
Writing Your First Program
Open a text editor (e.g., Notepad, Sublime Text, or Visual Studio Code).
Write your first MicroPython program:
import time
while True:
print("Hello, World!")
time.sleep(1)
This simple program will print “Hello, World!” to the console every second.
Save the file as ‘main.py’ and copy it onto your Raspberry Pi Pico’s drive (the ‘RPI-RP2’ drive).
The Pico will automatically run the program when you save it. You should see “Hello, World!” printed to the console every second.
Controlling GPIO Pins
One of the main features of the Raspberry Pi Pico is its general-purpose input/output (GPIO) pins. These pins can be used for reading sensors or controlling external devices. Here’s an example program that blinks an LED connected to GPIO pin 25:
import machine
import time
led = machine.Pin(25, machine.Pin.OUT) # set pin 25 as output
while True:
led.value(1) # turn on the LED
time.sleep(0.5) # wait for half a second
led.value(0) # turn off the LED
time.sleep(0.5) # wait for half a second
To run this program, save it as ‘main.py’ and copy it onto your Raspberry Pi Pico’s drive (the ‘RPI-RP2’ drive). The LED should now blink on and off every second.
Using Libraries
MicroPython has a rich ecosystem of libraries that can be used to add functionality to your projects. To install a library, you will need to download the UPy file from the library’s GitHub repository and copy it onto your Raspberry Pi Pico’s drive (the ‘RPI-RP2’ drive).
For example, let’s use the ‘micropython-adafruit-ssd1306’ library to display text on an OLED display:
Download the UPy file from https://github.com/micropython/micropython-adafruit-ssd1306
Copy ‘ssd1306.py’ and ‘framebuf.py’ onto your Raspberry Pi Pico’s drive (the ‘RPI-RP2’ drive).
Write the following program:
import ssd1306
from machine import I2C, Pin
import framebuf
i2c = I2C(0)
oled = ssd1306.SSD1306_I2C(128, 64, i2c, addr=0x3C)
oled.fill(0) # clear the display
oled.text("Hello, OLED!", 0, 0) # write text to the display
oled.show() # update the display
Save this program as ‘main.py’ and copy it onto your Raspberry Pi Pico’s drive (the ‘RPI-RP2’ drive). You should now see “Hello, OLED!” displayed on the OLED screen connected to your Pico.
Conclusion
Congratulations! You have successfully programmed a Raspberry Pi Pico using MicroPython. This is just the beginning of what you can do with this versatile microcontroller. As you continue learning, explore more libraries and experiment with different projects to unlock the full potential of your Raspberry Pi Pico.