Integrating DHT Sensors with Python and Raspberry Pi using adafruit_dht Library
A step-by-step guide to install the adafruit_dht library on your Raspberry Pi and get started with reading temperature and humidity data from a DHT sensor. …
Updated September 22, 2023
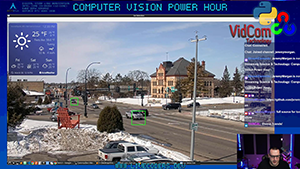
Need help with your Raspberry Pi?
Contact Me!
Do you love silly Raspberry Pi Projects?
Check out my this YouTube Channel!
A step-by-step guide to install the adafruit_dht library on your Raspberry Pi and get started with reading temperature and humidity data from a DHT sensor.
The Adafruit DHT series of sensors are simple, inexpensive, and easily integrated into your Raspberry Pi projects for measuring temperature and humidity. This article will guide you through the process of installing the adafruit_dht library on your Raspberry Pi and reading data from a DHT sensor using Python.
- Update your system: Before you begin, ensure that your Raspberry Pi is up-to-date by running these commands in the terminal:
sudo apt-get update
sudo apt-get upgrade
- Install the required dependencies: The adafruit_dht library requires some additional Python libraries to work properly. Run this command in your terminal to install them:
sudo pip3 install RPi.GPIO adafruit-blinka Adafruit-DHT
If you’re using Python 2, replace “pip3” with “pip”.
- Connect the DHT sensor: The DHT11, DHT222, and AM2302 sensors have three pins - VCC (connect to 3.3V power), GND (connect to ground), and DAT (data pin). Connect these pins to your Raspberry Pi according to the following diagram:
DHT Sensor | Raspberry Pi
----------- | --------------
+ | 1 (3.3V) Power
Out | 7 (GPIO4) Data
- | 9 (Ground)
Note: The DHT222 and AM2302 sensors are the same, but the AM2302 is more accurate.
- Install adafruit_dht: Run this command in your terminal to download the latest version of the library from GitHub:
git clone https://github.com/adafruit/Adafruit_Python_DHT.git
cd Adafruit_Python_DHT
sudo python3 setup.py install
If you’re using Python 2, replace “python3” with “python”.
- Test the sensor: To verify that everything is working correctly, create a new Python file (e.g.,
dht_test.py
) and add the following code:
import Adafruit_DHT
import time
# Set the pin number and sensor type (DHT11, DHT222, or AM2302)
sensor = Adafruit_DHT.DHT222
pin = 4
while True:
humidity, temperature = Adafruit_DHT.read_retry(sensor, pin)
if humidity is not None and temperature is not None:
print("Temperature: {:.1f}C Humidity: {:.1f}%".format(temperature, humidity))
else:
print("Failed to retrieve data from sensor")
time.sleep(2)
Save the file and run it using python3 dht_test.py
or python dht_test.py
, depending on your Python version. The script will continuously read data from the DHT sensor and print the temperature and humidity values to the terminal.
That’s it! You have successfully installed adafruit_dht on your Raspberry Pi and can now start building projects that use DHT sensors. For more information about the library, visit the official GitHub repository.