A step-by-step guide on connecting a camera module to your Raspberry Pi 3 B+
This article will explain how to connect a camera module to your Raspberry Pi 3 B+ and start capturing images using the Python programming language. …
Updated August 22, 2023
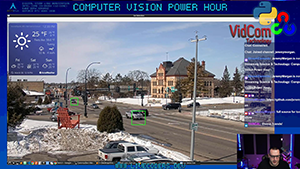
Need help with your Raspberry Pi?
Contact Me!
Do you love silly Raspberry Pi Projects?
Check out my this YouTube Channel!
This article will explain how to connect a camera module to your Raspberry Pi 3 B+ and start capturing images using the Python programming language.
- Preparation Before you begin, make sure you have the following items:
- Raspberry Pi 3 B+
- MicroSD card (8GB or higher) with Raspbian installed
- USB power supply
- Camera module compatible with the Raspberry Pi (e.g., Pi NoIR camera v2)
- HDMI monitor, mouse, and keyboard for initial setup
- Ethernet cable or WiFi adapter for network access
- Connecting the Camera Module Connect the camera module to the Raspberry Pi using the following pins:
- Pin 1 (3.3V power): Connect to pin 1 on the camera module
- Pin 2 (5V power): Leave unconnected
- Pin 3 (SDA): Connect to pin 3 on the camera module
- Pin 4 (5V power): Leave unconnected
- Pin 5 (Ground): Connect to pin 6 on the camera module
- Pin 6 (SCL): Connect to pin 5 on the camera module
- Pin 7 (GPIO4): Connect to pin 13 on the camera module (clock)
- Pin 8 (GPIO14): Connect to pin 12 on the camera module (data)
- Pin 9 (Ground): Connect to pin 10 on the camera module
- Pin 11 (GPIO15): Connect to pin 11 on the camera module (enable)
- Pin 12 (GPIO18): Leave unconnected
- Pin 13 (GPIO23): Connect to pin 15 on the camera module (reset)
- Pin 14 (GPIO24): Leave unconnected
- Pin 15 (GPIO25): Leave unconnected
- Pin 16 (GPIO8): Connect to pin 16 on the camera module (PWM)
- Pin 17: Connect to pin 19 on the camera module (FPS)
- Pin 18: Leave unconnected
- Pin 19 (Ground): Connect to pin 20 on the camera module
- Pin 20 (MOSI): Connect to pin 21 on the camera module
- Pin 21 (GPIO9): Connect to pin 22 on the camera module
- Pin 22 (MISO): Connect to pin 23 on the camera module
- Pin 23 (SCLK): Connect to pin 24 on the camera module
- Pin 24: Leave unconnected
- Pin 25: Connect to pin 7 on the camera module
- Pin 26: Connect to pin 11 on the camera module
- Enabling the Camera Module After connecting the camera, follow these steps to enable it in Raspbian:
- Start Raspberry Pi and log in with your username and password
- Open a terminal window by clicking on the “Terminal” icon on the top left menu bar
- Type
sudo raspi-config
and press Enter. This will open the Raspberry Pi configuration tool - Navigate to “Interfacing Options” and select “Camera”
- Choose “Enable” and then “Finish”
- Reboot your Raspberry Pi by typing
sudo reboot
in the terminal window and pressing Enter
- Testing the Camera Module Once the camera module is enabled, test it using the following commands:
- Type
raspistill -o image.jpg
to take a photo and save it as “image.jpg” in your home directory - Type
raspivid -o video.h264
to record a video and save it as “video.h264” in your home directory
- Capturing Images with Python
To capture images using Python, you need to install the picamera library by typing
sudo apt-get install python3-picamera
in the terminal window. Then, create a new Python file (e.g., camera.py) and add the following code:
from picamera import PiCamera
import time
camera = PiCamera()
camera.resolution = (1024, 768)
camera.start_preview()
time.sleep(5)
camera.capture('image.jpg')
camera.stop_preview()
This code will open the camera preview for 5 seconds and save an image named “image.jpg” in your home directory. You can modify the resolution by changing the values in camera.resolution
. To run the script, type python3 camera.py
in the terminal window.
- Recording Videos with Python To record videos using Python, create a new Python file (e.g., video.py) and add the following code:
from picamera import PiCamera
import time
camera = PiCamera()
camera.resolution = (1024, 768)
camera.start_recording('video.h264')
time.sleep(5)
camera.stop_recording()
This code will record a video for 5 seconds and save it as “video.h264” in your home directory. You can modify the recording time by changing the value in time.sleep
. To run the script, type python3 video.py
in the terminal window.
That’s it! You have successfully connected a camera to your Raspberry Pi 3 B+ and started capturing images and videos using Python. With these basic skills, you can build more advanced applications for image processing, object recognition, or surveillance systems.