Learn how to connect two Raspberry Pi’s using a simple Python script and GPIO pins
This article will show you step by step instructions on how to connect two Raspberry Pi’s together using a Python script and General Purpose Input/Output (GPIO) pins. It will also cover how to test th …
Updated September 23, 2023
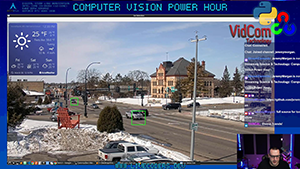
Need help with your Raspberry Pi?
Contact Me!
Do you love silly Raspberry Pi Projects?
Check out my this YouTube Channel!
This article will show you step by step instructions on how to connect two Raspberry Pi’s together using a Python script and General Purpose Input/Output (GPIO) pins. It will also cover how to test the connection between the two devices.
Requirements
- Two Raspberry Pi’s with Raspbian OS installed
- Breadboard
- Jumper wires
- LEDs
- Resistors (optional)
Step 1: Connecting the GPIO pins
First, we need to connect the two Raspberry Pi’s using the GPIO pins. Here are the connections you should make:
Raspberry Pi 1 | Raspberry Pi 2 |
---|---|
GND | GND |
GPIO4 | GPIO18 |
We’ll be using GPIO pin 4 on the first Raspberry Pi and GPIO pin 18 on the second one. Connect the GND pins together to provide a common ground for both devices.
Step 2: Setting up the Python script
Next, we need to create a simple Python script on each Raspberry Pi that will allow us to turn an LED on and off using GPIO pin 4. Here is the code for the first Raspberry Pi:
import RPi.GPIO as GPIO
import time
GPIO.setmode(GPIO.BCM)
GPIO.setup(4, GPIO.OUT)
while True:
GPIO.output(4, True) # turn on LED
time.sleep(1) # wait one second
GPIO.output(4, False) # turn off LED
time.sleep(1) # wait another second
And here is the code for the second Raspberry Pi:
import RPi.GPIO as GPIO
import time
GPIO.setmode(GPIO.BCM)
GPIO.setup(18, GPIO.IN, pull_up_down=GPIO.PUD_UP)
while True:
if not GPIO.input(18): # check if pin 18 is low (connected to pin 4 on first Pi)
print("Pin 18 is low")
else:
print("Pin 18 is high")
Save these scripts as led_on.py
and led_check.py
, respectively. You can use any text editor to create and save the files. For example, you can use nano
:
sudo nano led_on.py
# paste code, press Ctrl+X, Y, then Enter to save and exit
Step 3: Running the scripts
Now it’s time to run the Python scripts on each Raspberry Pi. Open two terminal windows - one for each device. On each window, navigate to the directory where you saved the script using cd
command:
cd /path/to/script/directory
Then, run the led_on.py
script on the first Raspberry Pi by typing:
sudo python3 led_on.py
And run the led_check.py
script on the second Raspberry Pi:
sudo python3 led_check.py
Step 4: Testing the connection
If everything is working correctly, you should see the LED on the first Raspberry Pi turn on and off every second, and the message “Pin 18 is low” printed in the terminal of the second Raspberry Pi whenever pin 18 is low (connected to pin 4 on the first Pi). This shows that there is a connection between the two devices.
Conclusion
Congratulations! You have successfully connected two Raspberry Pi’s together using a Python script and GPIO pins. With this basic setup, you can expand your project to include more complex communication between the devices or use different types of sensors and actuators to create a fully functional system. Remember that the possibilities are endless with Raspberry Pi’s!