Understanding the SPI Clock Speed of Your Raspberry Pi and Setting it Correctly
Learn how to check your Raspberry Pi’s SPI clock speed using both Python and command line interface, as well as how to set it to an optimal value for your project. …
Updated September 21, 2023
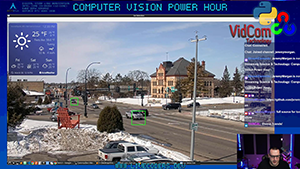
Need help with your Raspberry Pi?
Contact Me!
Do you love silly Raspberry Pi Projects?
Check out my this YouTube Channel!
Learn how to check your Raspberry Pi’s SPI clock speed using both Python and command line interface, as well as how to set it to an optimal value for your project. When working with Raspberry Pi and SPI (Serial Peripheral Interface), you may need to check the clock speed of your device. The SPI clock is responsible for controlling the communication between the master and slave devices, so having a good understanding of it is crucial for efficient data transfer. In this article, we will explore how to check the SPI clock speed using both Python and command line interface, as well as how to set it correctly for your project.
Checking Raspberry Pi SPI Clock Speed Using Python
Python provides a convenient way to check the SPI clock speed of your Raspberry Pi by using the spidev
library. First, make sure you have the library installed:
sudo apt-get install python3-spidev
Next, create a new Python script (e.g., check_spi_clock.py
) and add the following code to it:
import spidev
# Create SPI device
spi = spidev.SpiDev()
spi.open(0, 0)
# Get current SPI clock speed
current_speed = spi.max_speed_hz
print("Current SPI clock speed: {} Hz".format(current_speed))
Now run the script:
python3 check_spi_clock.py
This will output the current SPI clock speed of your Raspberry Pi in Hertz (Hz).
Checking Raspberry Pi SPI Clock Speed Using Command Line Interface
Alternatively, you can check the SPI clock speed using the command line interface by running the spi-tools
utility. First, make sure it is installed:
sudo apt-get install spi-tools
Next, run the following command to get the current SPI clock speed:
spi_view -r 0,0
This will output information about the SPI device and its settings, including the maximum clock speed. Look for a line that starts with “Max Speed” followed by the speed in Hz.
Setting Raspberry Pi SPI Clock Speed
Once you have determined your desired SPI clock speed, you can set it using either Python or command line interface. However, make sure to choose a value within the device’s capabilities and your project requirements for efficient data transfer.
Using Python:
import spidev
# Create SPI device
spi = spidev.SpiDev()
spi.open(0, 0)
# Set new SPI clock speed (e.g., 1 MHz)
new_speed = 10000000
spi.max_speed_hz = new_speed
print("New SPI clock speed: {} Hz".format(spi.max_speed_hz))
Using Command Line Interface:
spi_set_clock -d 0,0 -s 10000000
This will set the SPI clock speed to 1 MHz for bus 0 and device 0. Replace 0,0
with your desired bus and device number, and 10000000
with the desired speed in Hz.
In conclusion, understanding the SPI clock speed of your Raspberry Pi is crucial when working with SPI devices. You can check the current speed using either Python or command line interface, and set it to an optimal value for your project’s needs. Remember to choose a speed within the device’s capabilities and your project requirements for efficient data transfer.